The gradient color trend is extremely versatile.
Introduction
If you want to feel a different and modern look in your apps then the go for choice is gradient color. The blend of different shades of color, gradient creates a completely unique feelings to the modern designs. Companies such as ,Hulu, Spotify, Airbnb and Laracast often use gradient colors in their products.
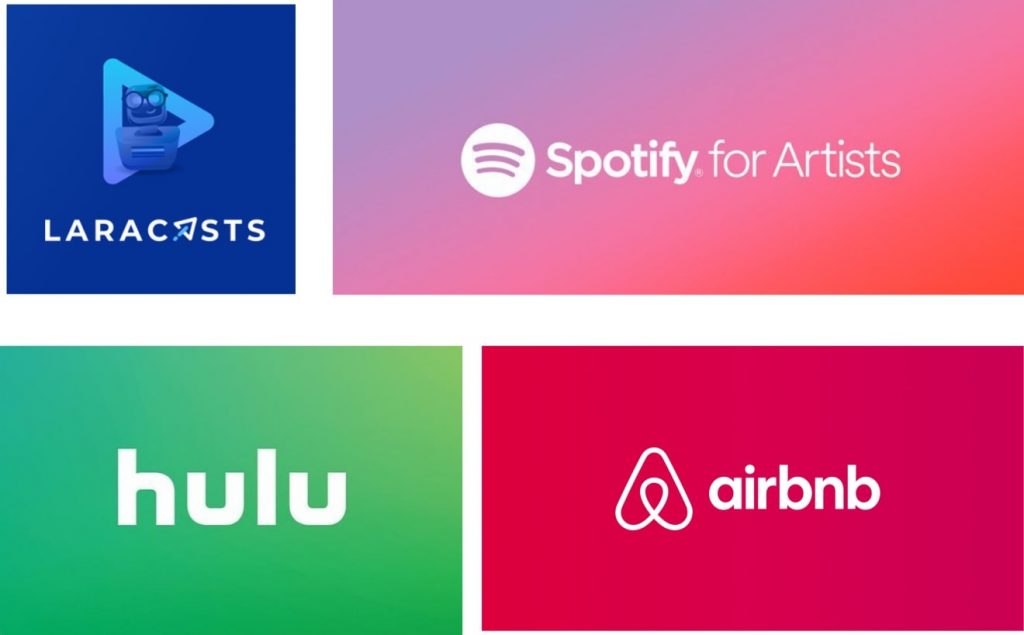
This article will teach you how to add and customize these gradient designs into your Flutter app. There are plugins for this to achieve, however we want to implement same exact feature without using any third-party plugins.
So lets begin…
Step 1 — Setting Up the Project
To create a new flutter application simply run the following command. Please make sure to complete the Prerequisites for any flutter project if you are running this command for the first time.
flutter create flutter_gradient_example
Navigate to the project directory you just created:
cd flutter_gradient_example
Step 2 — Using LinearGradient
Open main.dart with your code editor and modify the boilerplate code provide by flutter team. We write most of the our code on this file. We are using simple statelessWidget since there is not state management that we need to take care of.
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Gradient AppBar',
home: HomePage());
}
}
In the code below, you will notice we have used Scaffold and AppBar widgets. With these two widgets we are able to create an AppBar on top of our app.
Now use flexibleSpace property, so we can use a Container widget for a gradient layout. There are various kind of gradient but in this case we will use linear gradient method. It is also possible to have additional colors but in this example we are using only red and orange color.
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
// implement the app bar
appBar: AppBar(
title: const Text('Gradient AppBar'),
flexibleSpace: Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: [Colors.red, Colors.orange]),
),
),
),
);
}
}
Step 2 — Run the emulator
Compile your code and have it run in an emulator. You will see gradient AppBar on your app.
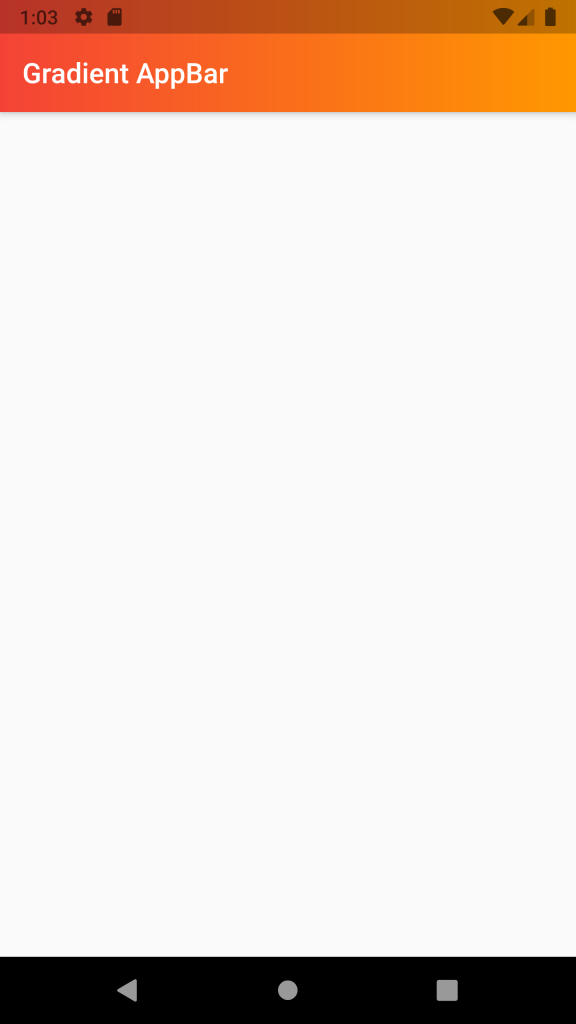
Thats it. Now you have achieved gradient AppBar in your flutter app. You can find full code in this Github repo.