Introduction
One of the very first reasons to get up early when you’re beachside is to see beautiful sunrise. Today I spent time on beach area aiming to complete this article I started last night. This is a short article where I wrote about Next.js navigation and its implementation. So without further ado let’s jump into the topic.
Websites and web applications generally have more than one pages. But you may wondering, how to achieve routing in Next.js so users able to navigate between the pages. In this article, we’ll explore how to navigate between the pages but before that, first let’s look into the brief introduction of Next.js.
What is Next.js ?
In our previous article we have discussed about Next.js and its cool features in more in-depth. Next.js is an open-source development framework built on top of React.js. It is React based framework having various functionalities to power up both server-side rendering and generating client side static websites.
Next.js gives you amazing developer experience with all the features it provides for any production ready application. Features such as hybrid static & server rendering, TypeScript support, smart bundling, route pre-fetching give developers a seamless development experience.
Now, let’s explore how to add more pages to our application and how to manage page routing in Next.js.
In this article, we will learn:
- Setup project in Next.js
- Create pages
- Use Link component to enable client-side navigation between pages.
1. Setup project in Next.js
We start with initial setup of the Next.js project. If this is the first time you are trying to create a project in Next.js then refer Nextjs official website where you get an extensive knowledge on Nextjs and its core functionalities.
To create a new app simply write the following command create-next-app, which sets up everything automatically for you. To create a project, run:
npx create-next-app@latest
# or
yarn create next-app
After installation is completed:
- Run
npm run dev
oryarn dev
to start the development server onhttp://localhost:3000
- Visit
http://localhost:3000
on your browser to view your application
2. Create pages
In Next.js, usually we create separate folder directory called pages. This folder consists of all the pages for the application. Pages are associated with the routes based on their file name. For example:
- pages/index.js is associated with the / route.
const Index = () => <h1>Home page!</h1>
export default Index;
- pages/about.js is associated with the /about.js route.
export default function About() {
return <h1>About</h1>
}
3. Link component
Now we use next/link to enable the redirection between pages. To link different pages, we use <a> HTML tag. Next.js uses Link Component from next/link to wrap the <a> tag. <Link> allows you to do client-side navigation to a different page in the application.
To use Nextjs <Link>
First, open pages/index.js, and import the Link component from next/link by adding this line at the top:
import Link from 'next/link'
export default function Home() {
return (
<div className="lg:inline-flex lg:flex-row lg:ml-auto lg:w-auto w-full
lg:items-center items-start flex flex-col lg:h-auto mr-12">
<Link href="/">
<a className="navtext uppercase">Home</a>
</Link>
<Link href="/about">
<a className="navtext uppercase">About</a>
</Link>
<Link href="/contact">
<a className="navtext uppercase"> Contact</a>
</Link>
</div>
);
}
In this example we create about us page only for simplicity. Therefore, open pages/about.js and start writing content. You can add rest of the pages later.
function about() {
return (
<div className="container mx-auto px-4 sm:px-12 py-12">
<h1 className="text-xl font-medium">About</h1>
<p className="text-md font-base py-12">
is simply dummy text of the printing and typesetting industry. Lorem
Ipsum has been the industry's standard dummy text ever since the 1500s,
when an unknown printer took a galley of type and scrambled it to make a
type specimen book. It has survived not only five centuries, but also
the leap into electronic typesetting, remaining essentially unchanged.
It was popularised in the 1960s with the release of Letraset sheets
containing Lorem Ipsum passages, and more recently with desktop
publishing software like Aldus PageMaker including versions of Lorem
Ipsum.
</p>
</div>
);
}
export default about;
Wrap Up
In this tutorial we learnt how to use Next.js link to navigate between different pages on the websites. Next.js comes with so many great features including Client-Side Navigation and Dynamic Navigation but in this example we have used client-side navigation using Link tag. Now linking pages in any Next.js website is easier than ever, Huge thanks to the Next.js team.
Demo
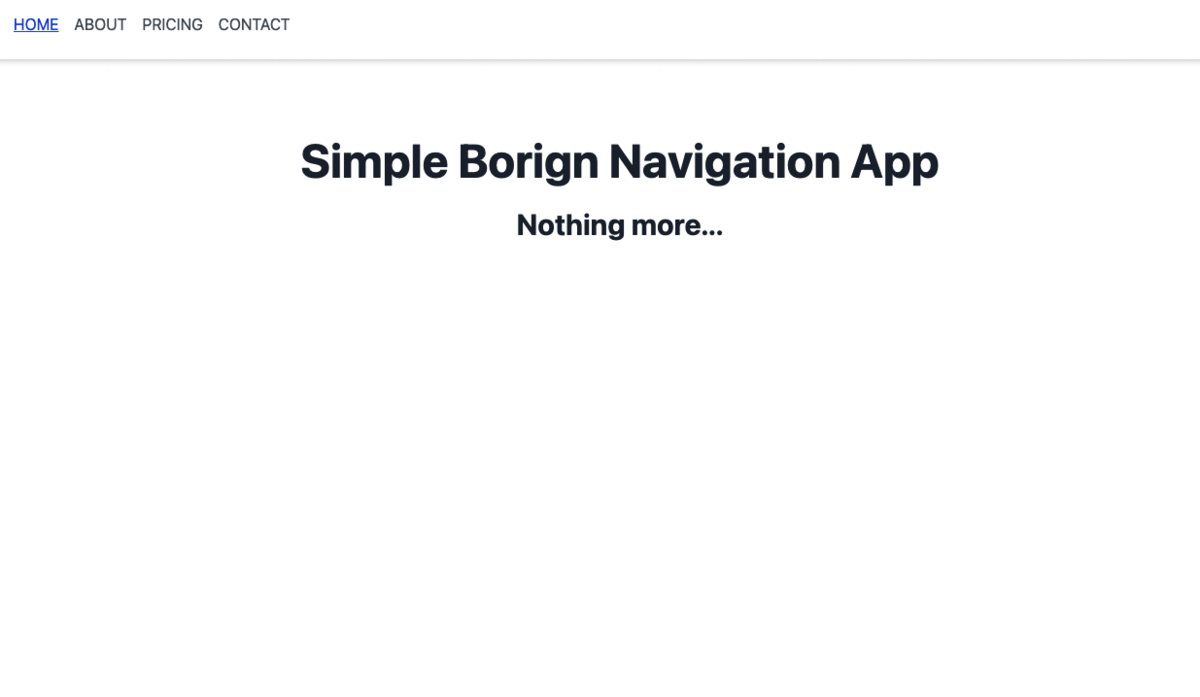
Full Code
You can get full code from my GitHub repository. Also this demo is deployed in Vercel. Have a look!