Sandbanks are everywhere here in Maldives. Today, I went to a sandbank called Vagaath gale hutta (I believe that is the correct location), which is roughly 5 miles north west of Dhevvadhoo. During the trip I took time to write this tutorial.
So without further ado let’s begin…
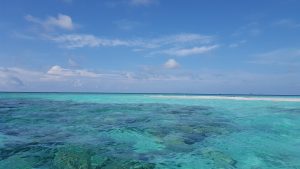
Front-end technologies are constantly evolving. Some technologies are becoming incredibly popular among the developers while others get less attraction. When it comes to front-end development, now React JS has become number one choice for most of the developers.
When it comes to CSS frameworks, TailwindCSS started to get more stars on Github meaning developers love to use TailwindCSS over other CSS technologies.
React JS
React JS is a JavaScript library developed by Facebook. React JS is efficient, declarative and flexible open-source JavaScript library which lets developers to build scalable frontend web applications much faster and easier. It offers tons of benefits. Compare to other front-end frameworks, React JS is easy to learn, SEO-friendly, helps to build rich user interfaces, able to write custom components and much more.
TailwindCSS
TailwindCSS is utility-based low level CSS framework intended to ease building web applications much faster and efficiently. TailwindCSS helps to build websites without ever leaving your HTML files.
In this tutorial, you will learn how to add tailwindCSS to an existing React JS application. So without further ado let’s create a react app by running the following command.
npx create-react-app react-tailwind
cd react-tailwind
Go to directory of your my-app and run the following command.
npm i tailwindcss postcss-cli autoprefixer -D
Enter the following command to create the default configuration. This will create a tailwind.js file in the current directory.
npx tailwind init tailwind.js --full
Now, we need to create postcss.config.js file. For that simply run the command below. PostCSS is a tool for transforming styles with JS plugins.
touch postcss.config.js
const tailwindcss = require('tailwindcss');
module.exports = {
plugins: [
tailwindcss('./tailwind.js'),
require('autoprefixer')
],
};
Create an assets directory in the src folder.
mkdir ./src/assets
Then create a file called tailwind.css in ./src/assets.
touch ./src/assets/tailwind.css
Now open tailwind.css and then import the following tailwind files as shown.
@import "tailwindcss/base";
@import "tailwindcss/components";
@import "tailwindcss/utilities";
Now it is time to modify package.json file just little bit. Simply add the following lines in the scripts tags.
"scripts": {
"start": "yarn watch:css && react-scripts start",
"build": "yarn build:css && react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject",
"build:css": "postcss src/assets/tailwind.css -o src/assets/main.css",
"watch:css": "postcss src/assets/tailwind.css -o src/assets/main.css"
},
Lastly import the file ./src/assets/main.css in the App.js file (or the root file of the application).
import React from "react";
import ReactDOM from "react-dom";
import './assets/main.css';
ReactDOM.render(<App />, document.getElementById("root"));
Now run the development server. Simply run:
npm start
Thats all! Now you can able to use Tailwind classes in your React JS application.
Code: Github