Introduction
Learning Flutter is a great idea. Flutter is great if you want to build a career in mobile app development. I’ve planned to write short series based articles on the things I learned about flutter.
In this article, you will explore how to change the overall look across your apps with Flutter themes.
Step 1 — Setting Up the Project
To create a new flutter application simply run the following command. Oh do not forget to complete the installation process of flutter SDK, its prerequisites and the environment setup.
flutter create flutter_custom_theme_example
Flutter create command will give us a demo application that will display a simple clickable button which increment the numbers as we click the button. Now navigate to the new project directory that we just have created.
cd flutter_custom_theme_example
We need to see how our code looks like on the simulators. For that simply run the following code below. We want to enable hot reloading so it reflects the changes as we make to our code.
open -a simulator
Now that you have a working boilerplate Flutter application. It is the time to add customization to the app.
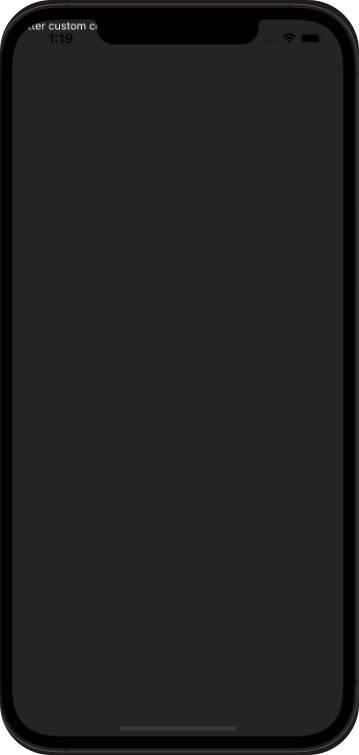
Step 2 — Customize Global Themes
Create a new folder named utils inside lib folder. Create a new file colors.dart inside utils.
import 'package:flutter/material.dart';
const mobileBackgroundColor = Color.fromRGBO(0, 0, 0, 1);
const mobileSearchColor = Color.fromRGBO(38, 38, 38, 1);
const blueColor = Color.fromRGBO(0, 149, 246, 1);
const primaryColor = Colors.white;
const secondaryColor = Colors.grey;
Now we have created a color style globally for our application which can be used anywhere in our app. Open main.dart file and remove all the code given by flutter by default.
We want add dark theme to our app, therefore add dark theme using themeData given by flutter. Next add Scaffold widget to the main.dart file. Now we can see it has changed to default dark color provided by flutter team.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Custom Color Example',
theme: ThemeData.dark(),
home: Scaffold(body: Text('Flutter custom color example')),
);
}
}
We want a different colors, so this is the point we want to use colors.dart file created earlier so that we can use our own dark theme colors. Import colors.dart file inside our main.dart file. Then add our custom color to themeData shown below code.
import 'package:flutter/material.dart';
import 'package:flutter_custom_theme_example/colors.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Custom Color Example',
theme: ThemeData.dark().copyWith(
scaffoldBackgroundColor: mobileBackgroundColor,
primaryColor: secondaryColor),
home: Scaffold(
appBar: AppBar(
title: const Text('Flutter custom color example'),
),
body: Center(
child: Text(
'Flutter custom color example',
)),
),
);
}
}
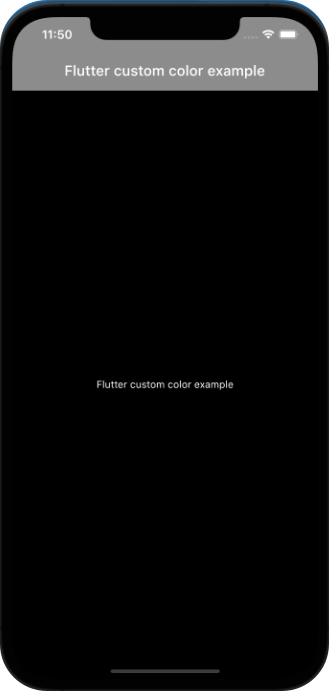
Thats it! Now we have added our own custom colors to the flutter app. Yes this is very basic styling however in future tutorial we can add more styles and colors to flutter apps. You can find full code in this Github repo.